高レベルの API v2
注
これは 新しい試験 的モジュールで、あるバージョンから次のバージョンに変更される可能性があります。 執筆時点では、高レベルの API v2 は、以前の API のすべての機能を実装するわけではありません。
ロケーション ライブラリ の高レベルの API v2 では 、さまざまな種類のパス・マッチャーをインスタンス化する方法と 、以下のインターフェイスを使用してアルゴリズムを直接実装するためのファクトリメソッドが提供されています。
-
ProximitySearch
座標からトポロジーセグメント(頂点)を検索します。
-
DirectedGraph
効率的なデータ構造でトポロジをナビゲートします。
-
PropertyMap
道路、ナビゲーション、高度なナビゲーション、および ADAS 属性にアクセスします。
API には、異なるカタログバージョン間で共有されているブロブを再利用できる新しいキャッシュメカニズムも導入されています。 これにより、前のアプローチと比較してメモリ使用量を削減できます。 除去方式はカタログベースで定義され、今後使用されなくなったレイヤーの削除を許可します。
設定中です
API の使用を開始する前に location-integration-optimized-map-dcl2
、次の依存関係を追加する必要があります。
libraryDependencies ++= Seq(
"com.here.platform.location" %% "location-integration-optimized-map-dcl2" % "0.21.788"
)
<dependencies>
<dependency>
<groupId>com.here.platform.location</groupId>
<artifactId>location-integration-optimized-map-dcl2_${scala.compat.version}</artifactId>
<version>0.21.788</version>
</dependency>
</dependencies>
dependencies {
compile group: 'com.here.platform.location', name: 'location-integration-optimized-map-dcl2_2.12', version:'0.21.788'
}
位置情報ライブラリ用に最適化された地図のバージョンを開きます
まず、 OptimizedMapLayers のインスタンスを作成する必要があります。これは、すべてのアルゴリズムのファクトリで必要なものです。 BaseClient
インスタンスに基づいてこれを行うことができます。
import com.here.platform.data.client.base.scaladsl.BaseClient
import com.here.platform.location.integration.optimizedmap.OptimizedMapLayers
import com.here.platform.location.integration.optimizedmap.dcl2.OptimizedMapCatalog
val baseClient = BaseClient()
val optimizedMap: OptimizedMapLayers =
OptimizedMapCatalog.from(optimizedMapHRN).usingBaseClient(baseClient).newInstance.version(42)
import com.here.platform.data.client.base.javadsl.BaseClient;
import com.here.platform.data.client.base.javadsl.BaseClientJava;
import com.here.platform.location.integration.optimizedmap.OptimizedMapLayers;
import com.here.platform.location.integration.optimizedmap.dcl2.javadsl.OptimizedMapCatalog;
BaseClient baseClient = BaseClientJava.instance();
OptimizedMapLayers optimizedMap =
OptimizedMapCatalog.from(optimizedMapHRN)
.usingBaseClient(baseClient)
.newInstance()
.version(42);
近接検索
現実の場所をルーティンググラフの頂点に関連付けるため に、 ProximitySearch では、マップ上の特定のポイントから一定の距離内にある頂点を検索できます。
ファクトリクラスの ProximitySearches を使用して、ProximitySearch インスタンスを作成できます。
import com.here.platform.location.core.geospatial._
import com.here.platform.location.inmemory.graph.Vertex
import com.here.platform.location.integration.optimizedmap.geospatial.ProximitySearches
val proximitySearches: ProximitySearches = ProximitySearches(optimizedMap)
val proximitySearch: ProximitySearch[Vertex] = proximitySearches.vertices
val brandenburgerTor: GeoCoordinate = GeoCoordinate(52.516268, 13.377700)
val projections: Iterable[ElementProjection[Vertex]] =
proximitySearch.search(center = brandenburgerTor, radiusInMeters = 100)
import com.here.platform.location.core.geospatial.ElementProjection;
import com.here.platform.location.core.geospatial.GeoCoordinate;
import com.here.platform.location.core.geospatial.javadsl.ProximitySearch;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.geospatial.javadsl.ProximitySearches;
ProximitySearches proximitySearches = new ProximitySearches(optimizedMap);
ProximitySearch<GeoCoordinate, Vertex> proximitySearch = proximitySearches.vertices();
GeoCoordinate brandenburgerTor = new GeoCoordinate(52.516268, 13.377700);
Iterable<ElementProjection<Vertex>> projections = proximitySearch.search(brandenburgerTor, 100);
頂点のジオメトリは必ずしも線形ではないので、場合によってはジオメトリの各セグメント(連続するポイントのペア間のセグメント)について 1 つの ElementProjection を取得することがあります。
注
次の画像は、からの結果を示し ProximitySearches.vertices
ています。 検索では、各頂点に最も近い点のみが返されます。 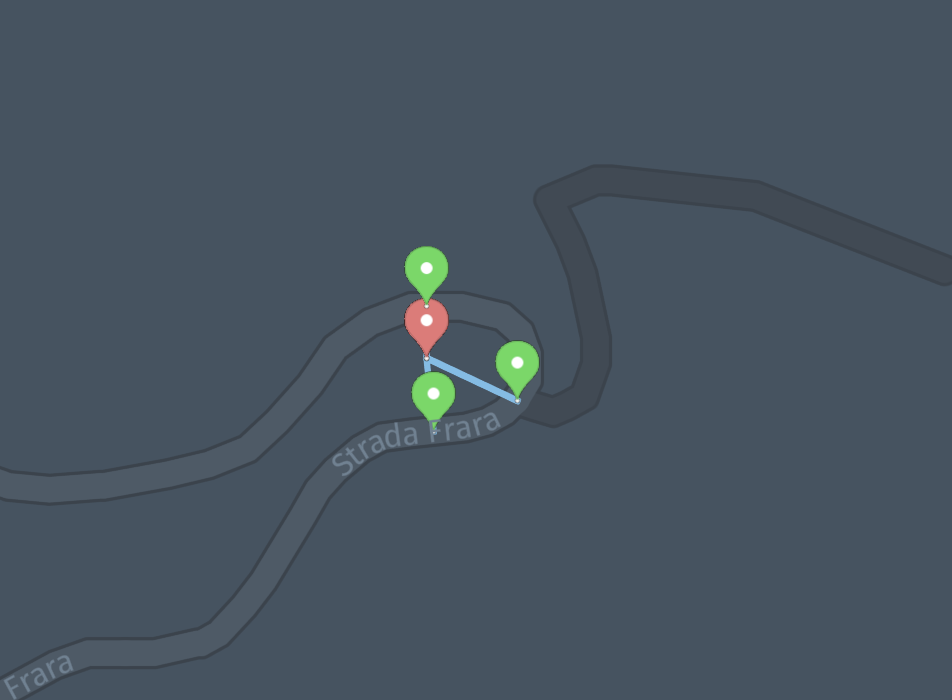
ProximitySearches.vertexGeometrySegments
バリアントを使用すると、頂点ごとに複数の投影を取得できます。 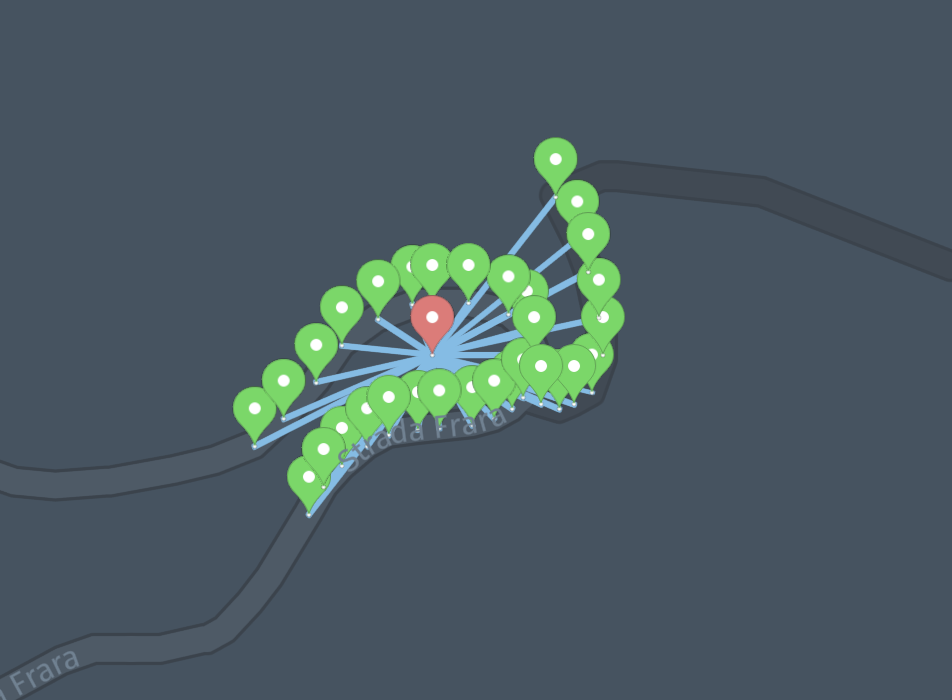
グラフ
グラフの抽象化を使用して、 HERE Map Content の道路トポロジおよびジオメトリをナビゲートできます。 グラフの各頂点は、ある方向の道路(トポロジセグメント)を表し、各エッジは、「ルーティンググラフ」セクションで説明されているように、道路間の物理的な接続を表します。
DirectedGraph インスタンスを作成するには、そのファクトリクラス Graphs を使用できます。
import com.here.platform.location.core.graph.DirectedGraph
import com.here.platform.location.inmemory.graph.{Edge, Vertex}
import com.here.platform.location.integration.optimizedmap.graph.Graphs
val graphs: Graphs = Graphs(optimizedMap)
val graph: DirectedGraph[Vertex, Edge] = graphs.forward
val outEdges: Iterator[Edge] = graph.outEdgeIterator(vertex)
import com.here.platform.location.core.graph.javadsl.DirectedGraph;
import com.here.platform.location.inmemory.graph.Edge;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.graph.javadsl.Graphs;
Graphs graphs = new Graphs(optimizedMap);
DirectedGraph<Vertex, Edge> graph = graphs.forward();
Iterator<Edge> outEdges = graph.getOutEdgeIterator(vertex);
注
道路トポロジセグメントには、物理的な進行方向ごとに 1 つずつ、常に 2 つの頂点が関連付けられています。 PropertyMaps を使用して属性を収集し、決定を下すことが PropertyMaps.roadAccess
できます。たとえば、以下で説明するように、さまざまな輸送モードの進行方向に関する情報を取得できます。
パスマッチャー
記録された地理座標のシーケンスを道路トポロジグラフのパスに解決するには、パスマッチャーを使用します。 パスマッチャーは、各入力ポイントに最も近い可能性が高い道路上の位置、およびポイント間の最も可能性の高い接続(遷移)についての情報を返します。
図 1. パスの一致 注
プローブデータのデンスおよびスパーストレース
記録された地理座標のシーケンスは 、トレースまたはプローブトレースと呼ばれます。 パス・ \'83\'7d ッシャーは、デンス・トレースとスパース・トレースを区別します。 密なトレースは、地図内の道路セグメントの長さと比較して短い連続するプローブ間の距離によって特徴付けられます。 通常、 1 ~ 5 秒ごとに収集されたプローブは密度が高いと見なされます。
ファクトリクラスの PathMatcher を使用して、PathMatcher インスタンスを作成できます。
import com.here.platform.location.core.geospatial.GeoCoordinate
import com.here.platform.location.core.mapmatching.{MatchedPath, PathMatcher}
import com.here.platform.location.inmemory.graph.Vertex
import com.here.platform.location.integration.optimizedmap.mapmatching.PathMatchers
val pathMatchers = PathMatchers(optimizedMap)
val pathMatcher: PathMatcher[GeoCoordinate, Vertex, Seq[Vertex]] =
pathMatchers.carPathMatcherWithTransitions
val matchedPath: MatchedPath[Vertex, Seq[Vertex]] = pathMatcher.matchPath(Seq(coordinates))
import com.here.platform.location.core.geospatial.javadsl.GeoCoordinateHolder;
import com.here.platform.location.core.mapmatching.javadsl.MatchedPath;
import com.here.platform.location.core.mapmatching.javadsl.PathMatcher;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.mapmatching.javadsl.PathMatchers;
PathMatchers pathMatchers = new PathMatchers(optimizedMap);
PathMatcher<GeoCoordinateHolder, Vertex, List<Vertex>> pathMatcher =
pathMatchers.carPathMatcherWithTransitions();
MatchedPath<Vertex, List<Vertex>> matchedPath =
pathMatcher.matchPath(Arrays.asList(coordinates));
パス \'83\'7d ネージャを作成するには、次のようなさまざまな方法を使用できます。
-
は unrestrictedPathMatcherWithoutTransitions
、走行制限から独立した結果を生成するマップマッチャーを作成し、高密度 GPS トレース( 5 秒ごとに少なくとも 1 つのポイント)に適しています。
は unrestrictedPathMatcherWithoutTransitions
、高密度プローブおよびすべての車両タイプについて、おそらく最もドライブされている経路に一致させる最も効果的な方法です。 運転によってこの経路をたどることは必ずしもできませんが、結果を使用して運転エラーについての警告を得ることができます。
は unrestrictedPathMatcherWithoutTransitions
、連続するポイントが直接接続されたトポロジセグメントにない場合、切断を引き起こすことがあります。これは、遷移の計算にルーティングアルゴリズムを使用しないためです。
unrestrictedPathMatcherWithTransitions
この変数を使用すると、単純な最短パスアルゴリズムで点を再結合するための作業がより大きくなります。
-
は carPathMatcherWithTransitions
、ほとんどの車の運転制限を考慮しています。また、車およびスパースデータ用に特別に設定されたパスマッチャーですが、高密度データにも対応します。 たとえば、このパスマッチャーは roadAccess
、プロパティに従って車によってアクセス可能な頂点にのみ入力ポイントを照合します。
carPathMatcherWithTransitions
は 、スパースデータのunrestrictedPathMatcherWithTransitions
場合よりも適切に動作します。これは、道路アクセスおよび曲がる際の制約を考慮した最短パスアルゴリズムを使用して、ポイント間をルーティングするためです。 状況によっては、連続するポイントの距離が遠すぎると(オンロード距離が約 30 km を超える)、到達不能と見なさ Unknown
れ、いずれかのポイントをマッチングできます。
carPathMatcherWithoutTransition
この変数は似ていますが、高密度データにのみ適しています。高密度データでは、連続するプローブ間の距離がマップ内の頂点の長さと比較して短くなります。
注
パス・マッチャーの出力について詳しく は、 API ガイドを参照してください。
プロパティマップ
ロケーション ライブラリ には、多くの地図機能にアクセスできるプロパティマップが用意されています。
PropertyMap インスタンスを作成するには、ファクトリクラス の PropertyMaps を使用し ます。また、頂点の長さなどを取得できます。
import com.here.platform.location.core.graph._
import com.here.platform.location.inmemory.graph.Vertex
import com.here.platform.location.integration.optimizedmap.graph.PropertyMaps
val propertyMaps = PropertyMaps(optimizedMap)
val length: PropertyMap[Vertex, Double] = propertyMaps.length
val lengthOfAVertex: Double = length(vertex)
import com.here.platform.location.core.graph.javadsl.PropertyMap;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.graph.javadsl.PropertyMaps;
PropertyMaps propertyMaps = new PropertyMaps(optimizedMap);
PropertyMap<Vertex, Double> length = propertyMaps.length();
Double lengthOfAVertex = length.get(vertex);
頂点の長さを取得するのに加えて、次の操作を行うこともできます。
-
HERE Map Content 参照と Optimized Map for Location Library の頂点を変換します
-
頂点に関連付けられている形状を取得します
-
頂点上の位置が特定の車両タイプにアクセスできるかどうかを確認します
-
通常、頂点を通過するトラフィックの速度を取得します(フリーフロー速度)
-
特定の車両タイプについて、特定の端部で示されている回転を制限するかどうかを指定します
-
などの道路属性を取得します
import com.here.platform.location.core.graph.RangeBasedProperty
import com.here.platform.location.integration.optimizedmap.graph.PropertyMaps
import com.here.platform.location.integration.optimizedmap.roadattributes._
val roadAttributes = PropertyMaps(optimizedMap).roadAttributes
val functionalClasses: Seq[RangeBasedProperty[FunctionalClass]] =
roadAttributes.functionalClass(vertex)
val overpassUnderpasses: Seq[RangeBasedProperty[OverpassUnderpass]] =
roadAttributes.overpassUnderpass(vertex)
val officialCountryCodes: Seq[RangeBasedProperty[OfficialCountryCode]] =
roadAttributes.officialCountryCode(vertex)
val physicalAttributes: Seq[RangeBasedProperty[PhysicalAttribute]] =
roadAttributes.physicalAttribute(vertex)
val roadClasses: Seq[RangeBasedProperty[RoadClass]] = roadAttributes.roadClass(vertex)
val specialTrafficAreaCategories: Seq[RangeBasedProperty[SpecialTrafficAreaCategory]] =
roadAttributes.specialTrafficAreaCategory(vertex)
val userDefinedCountryCodes: Seq[RangeBasedProperty[UserDefinedCountryCode]] =
roadAttributes.userDefinedCountryCode(vertex)
import com.here.platform.location.core.graph.javadsl.RangeBasedProperty;
import com.here.platform.location.core.graph.javadsl.RangeBasedPropertyMap;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.graph.javadsl.PropertyMaps;
import com.here.platform.location.integration.optimizedmap.roadattributes.*;
PropertyMaps.RoadAttributes roadAttributes = new PropertyMaps(optimizedMap).roadAttributes();
RangeBasedPropertyMap<Vertex, FunctionalClass> functionalClass =
roadAttributes.functionalClass();
List<RangeBasedProperty<FunctionalClass>> functionalClasses = functionalClass.get(vertex);
RangeBasedPropertyMap<Vertex, OverpassUnderpass> overpassUnderpass =
roadAttributes.overpassUnderpass();
List<RangeBasedProperty<OverpassUnderpass>> overpassUnderpasses = overpassUnderpass.get(vertex);
RangeBasedPropertyMap<Vertex, OfficialCountryCode> officialCountryCode =
roadAttributes.officialCountryCode();
List<RangeBasedProperty<OfficialCountryCode>> officialCountryCodes =
officialCountryCode.get(vertex);
RangeBasedPropertyMap<Vertex, PhysicalAttribute> physicalAttribute =
roadAttributes.physicalAttribute();
List<RangeBasedProperty<PhysicalAttribute>> physicalAttributes = physicalAttribute.get(vertex);
RangeBasedPropertyMap<Vertex, RoadClass> roadClass = roadAttributes.roadClass();
List<RangeBasedProperty<RoadClass>> roadClasses = roadClass.get(vertex);
RangeBasedPropertyMap<Vertex, SpecialTrafficAreaCategory> specialTrafficAreaCategory =
roadAttributes.specialTrafficAreaCategory();
List<RangeBasedProperty<SpecialTrafficAreaCategory>> specialTrafficAreaCategories =
specialTrafficAreaCategory.get(vertex);
List<RangeBasedProperty<UserDefinedCountryCode>> userDefinedCountryCodes =
roadAttributes.userDefinedCountryCode().get(vertex);
-
などのナビゲーション属性を取得します
import com.here.platform.location.core.graph.RangeBasedProperty
import com.here.platform.location.integration.optimizedmap.commonattributes.SpeedLimit
import com.here.platform.location.integration.optimizedmap.graph.PropertyMaps
import com.here.platform.location.integration.optimizedmap.navigationattributes._
val navigationAttributes = PropertyMaps(optimizedMap).navigationAttributes
val intersectionInternalCategories: Seq[RangeBasedProperty[IntersectionInternalCategory]] =
navigationAttributes.intersectionInternalCategory(vertex)
val laneCategories: Seq[RangeBasedProperty[LaneCategory]] =
navigationAttributes.laneCategory(vertex)
val throughLaneCounts: Seq[RangeBasedProperty[Int]] =
navigationAttributes.throughLaneCount(vertex)
val physicalLaneCounts: Seq[RangeBasedProperty[Int]] =
navigationAttributes.physicalLaneCount(vertex)
val localRoads: Seq[RangeBasedProperty[LocalRoad]] =
navigationAttributes.localRoad(vertex)
val roadUsages: Seq[RangeBasedProperty[RoadUsage]] =
navigationAttributes.roadUsage(vertex)
val lowMobilities: Seq[RangeBasedProperty[LowMobility]] =
navigationAttributes.lowMobility(vertex)
val roadDividers: Seq[RangeBasedProperty[RoadDivider]] =
navigationAttributes.roadDivider(vertex)
val speedCategories: Seq[RangeBasedProperty[SpeedCategory]] =
navigationAttributes.speedCategory(vertex)
val speedLimit: Seq[RangeBasedProperty[SpeedLimit]] =
navigationAttributes.speedLimit(vertex)
val supplementalGeometries: Seq[RangeBasedProperty[SupplementalGeometry]] =
navigationAttributes.supplementalGeometry(vertex)
val travelDirection: Seq[RangeBasedProperty[TravelDirection]] =
navigationAttributes.travelDirection(vertex)
val urban: Seq[RangeBasedProperty[Boolean]] =
navigationAttributes.urban(vertex)
val specialExplication: Option[SpecialExplication] =
navigationAttributes.specialExplication(edge)
val throughRoute: Option[ThroughRoute] =
navigationAttributes.throughRoute(edge)
val tmcCodes: Seq[RangeBasedProperty[Set[TrafficMessageChannelCode]]] =
navigationAttributes.trafficMessageChannelCodes(vertex)
import com.here.platform.location.core.graph.javadsl.PropertyMap;
import com.here.platform.location.core.graph.javadsl.RangeBasedProperty;
import com.here.platform.location.core.graph.javadsl.RangeBasedPropertyMap;
import com.here.platform.location.inmemory.graph.Edge;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.commonattributes.SpeedLimit;
import com.here.platform.location.integration.optimizedmap.graph.javadsl.PropertyMaps;
import com.here.platform.location.integration.optimizedmap.navigationattributes.*;
PropertyMaps.NavigationAttributes navigationAttributes =
new PropertyMaps(optimizedMap).navigationAttributes();
RangeBasedPropertyMap<Vertex, IntersectionInternalCategory> intersectionInternalCategory =
navigationAttributes.intersectionInternalCategory();
List<RangeBasedProperty<IntersectionInternalCategory>> intersectionInternalCategories =
intersectionInternalCategory.get(vertex);
RangeBasedPropertyMap<Vertex, LaneCategory> laneCategory = navigationAttributes.laneCategory();
List<RangeBasedProperty<LaneCategory>> laneCategories = laneCategory.get(vertex);
RangeBasedPropertyMap<Vertex, RoadUsage> roadUsage = navigationAttributes.roadUsage();
List<RangeBasedProperty<RoadUsage>> roadUsages = roadUsage.get(vertex);
RangeBasedPropertyMap<Vertex, Integer> throughLaneCount =
navigationAttributes.throughLaneCount();
List<RangeBasedProperty<Integer>> throughLaneCounts = throughLaneCount.get(vertex);
RangeBasedPropertyMap<Vertex, Integer> physicalLaneCount =
navigationAttributes.physicalLaneCount();
List<RangeBasedProperty<Integer>> physicalLaneCounts = physicalLaneCount.get(vertex);
RangeBasedPropertyMap<Vertex, LocalRoad> localRoad = navigationAttributes.localRoad();
List<RangeBasedProperty<LocalRoad>> localRoads = localRoad.get(vertex);
RangeBasedPropertyMap<Vertex, LowMobility> lowMobility = navigationAttributes.lowMobility();
List<RangeBasedProperty<LowMobility>> lowMobilities = lowMobility.get(vertex);
RangeBasedPropertyMap<Vertex, RoadDivider> roadDivider = navigationAttributes.roadDivider();
List<RangeBasedProperty<RoadDivider>> roadDividers = roadDivider.get(vertex);
RangeBasedPropertyMap<Vertex, SpeedCategory> speedCategory =
navigationAttributes.speedCategory();
List<RangeBasedProperty<SpeedCategory>> speedCategories = speedCategory.get(vertex);
RangeBasedPropertyMap<Vertex, SpeedLimit> speedLimit = navigationAttributes.speedLimit();
List<RangeBasedProperty<SpeedLimit>> speedLimits = speedLimit.get(vertex);
RangeBasedPropertyMap<Vertex, SupplementalGeometry> supplementalGeometry =
navigationAttributes.supplementalGeometry();
List<RangeBasedProperty<SupplementalGeometry>> supplementalGeometries =
supplementalGeometry.get(vertex);
RangeBasedPropertyMap<Vertex, TravelDirection> travelDirection =
navigationAttributes.travelDirection();
List<RangeBasedProperty<TravelDirection>> travelDirections = travelDirection.get(vertex);
RangeBasedPropertyMap<Vertex, Boolean> urban = navigationAttributes.urban();
List<RangeBasedProperty<Boolean>> urbans = urban.get(vertex);
PropertyMap<Edge, Optional<SpecialExplication>> specialExplication =
navigationAttributes.specialExplication();
Optional<SpecialExplication> specialExplications = specialExplication.get(edge);
PropertyMap<Edge, Optional<ThroughRoute>> throughRoute = navigationAttributes.throughRoute();
Optional<ThroughRoute> throughRoutes = throughRoute.get(edge);
RangeBasedPropertyMap<Vertex, Set<TrafficMessageChannelCode>> trafficMessageChannelCodes =
navigationAttributes.trafficMessageChannelCodes();
List<RangeBasedProperty<Set<TrafficMessageChannelCode>>> tmcCodes =
trafficMessageChannelCodes.get(vertex);
-
などの高度なナビゲーション属性を取得します
import com.here.platform.location.core.graph.{PointBasedProperty, RangeBasedProperty}
import com.here.platform.location.integration.optimizedmap.advancednavigationattributes._
import com.here.platform.location.integration.optimizedmap.commonattributes.SpeedLimit
import com.here.platform.location.integration.optimizedmap.graph.PropertyMaps
val advancedNavigationAttributes =
PropertyMaps(optimizedMap).advancedNavigationAttributes
val railwayCrossings: Seq[PointBasedProperty[RailwayCrossing]] =
advancedNavigationAttributes.railwayCrossing(vertex)
val speedLimit: Seq[RangeBasedProperty[SpeedLimit]] =
advancedNavigationAttributes.speedLimit(vertex)
val gradeCategory: Seq[RangeBasedProperty[GradeCategory]] =
advancedNavigationAttributes.gradeCategory(vertex)
val scenic: Seq[RangeBasedProperty[Scenic]] =
advancedNavigationAttributes.scenic(vertex)
import com.here.platform.location.core.graph.javadsl.PointBasedProperty;
import com.here.platform.location.core.graph.javadsl.PointBasedPropertyMap;
import com.here.platform.location.core.graph.javadsl.RangeBasedProperty;
import com.here.platform.location.core.graph.javadsl.RangeBasedPropertyMap;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.advancednavigationattributes.GradeCategory;
import com.here.platform.location.integration.optimizedmap.advancednavigationattributes.RailwayCrossing;
import com.here.platform.location.integration.optimizedmap.advancednavigationattributes.Scenic;
import com.here.platform.location.integration.optimizedmap.commonattributes.SpeedLimit;
import com.here.platform.location.integration.optimizedmap.graph.javadsl.PropertyMaps;
PropertyMaps.AdvancedNavigationAttributes advancedNavigationAttributes =
new PropertyMaps(optimizedMap).advancedNavigationAttributes();
PointBasedPropertyMap<Vertex, RailwayCrossing> railwayCrossing =
advancedNavigationAttributes.railwayCrossing();
List<PointBasedProperty<RailwayCrossing>> railwayCrossings = railwayCrossing.get(vertex);
RangeBasedPropertyMap<Vertex, SpeedLimit> speedLimit =
advancedNavigationAttributes.speedLimit();
List<RangeBasedProperty<SpeedLimit>> speedLimits = speedLimit.get(vertex);
RangeBasedPropertyMap<Vertex, GradeCategory> gradeCategory =
advancedNavigationAttributes.gradeCategory();
List<RangeBasedProperty<GradeCategory>> gradeCategories = gradeCategory.get(vertex);
RangeBasedPropertyMap<Vertex, Scenic> scenic = advancedNavigationAttributes.scenic();
List<RangeBasedProperty<Scenic>> scenics = scenic.get(vertex);
-
などの ADAS 属性を取得します
import com.here.platform.location.core.graph.{PointBasedProperty, RangeBasedProperty}
import com.here.platform.location.integration.optimizedmap.adasattributes._
import com.here.platform.location.integration.optimizedmap.graph.PropertyMaps
val adasAttributes = PropertyMaps(optimizedMap).adasAttributes
val builtUpAreaRoad: Seq[RangeBasedProperty[BuiltUpAreaRoad]] =
adasAttributes.builtUpAreaRoad(vertex)
val linkAccuracy: Seq[RangeBasedProperty[Int]] = adasAttributes.linkAccuracy(vertex)
val slope: Seq[PointBasedProperty[Slope]] = adasAttributes.slope(vertex)
val curvatureHeading: Seq[PointBasedProperty[CurvatureHeading]] =
adasAttributes.curvatureHeading(vertex)
val edgeCurvatureHeading: Option[CurvatureHeading] =
adasAttributes.edgeCurvatureHeading(edge)
val elevation: Seq[PointBasedProperty[Elevation]] =
adasAttributes.elevation(vertex)
import com.here.platform.location.core.graph.javadsl.*;
import com.here.platform.location.inmemory.graph.Edge;
import com.here.platform.location.inmemory.graph.Vertex;
import com.here.platform.location.integration.optimizedmap.adasattributes.BuiltUpAreaRoad;
import com.here.platform.location.integration.optimizedmap.adasattributes.CurvatureHeading;
import com.here.platform.location.integration.optimizedmap.adasattributes.Elevation;
import com.here.platform.location.integration.optimizedmap.adasattributes.Slope;
import com.here.platform.location.integration.optimizedmap.graph.javadsl.PropertyMaps;
PropertyMaps.AdasAttributes adasAttributes = new PropertyMaps(optimizedMap).adasAttributes();
RangeBasedPropertyMap<Vertex, BuiltUpAreaRoad> builtUpAreaRoad =
adasAttributes.builtUpAreaRoad();
List<RangeBasedProperty<BuiltUpAreaRoad>> builtUpAreaRoads = builtUpAreaRoad.get(vertex);
RangeBasedPropertyMap<Vertex, Integer> linkAccuracy = adasAttributes.linkAccuracy();
List<RangeBasedProperty<Integer>> linkAccuracies = linkAccuracy.get(vertex);
PointBasedPropertyMap<Vertex, Slope> slope = adasAttributes.slope();
List<PointBasedProperty<Slope>> slopes = slope.get(vertex);
PointBasedPropertyMap<Vertex, CurvatureHeading> curvatureHeading =
adasAttributes.curvatureHeading();
List<PointBasedProperty<CurvatureHeading>> curvatureHeadings = curvatureHeading.get(vertex);
PropertyMap<Edge, Optional<CurvatureHeading>> edgeCurvatureHeading =
adasAttributes.edgeCurvatureHeading();
Optional<CurvatureHeading> edgeCurvatureHeadings = edgeCurvatureHeading.get(edge);
PointBasedPropertyMap<Vertex, Elevation> elevation = adasAttributes.elevation();
List<PointBasedProperty<Elevation>> elevations = elevation.get(vertex);
基本的なキャッシュ設定およびキャッシュ統計情報
OptimizedMapCatalog を使用すると、ブロブキャッシュで使用されるバイト数およびメタデータ キャッシュで使用されるエントリ数を設定できます。 メタデータ キャッシュエントリの数が指定されていない場合は、ブロブキャッシュサイズに基づいた適切なデフォルト値が使用されます。
キャッシュにデータがロードされると、いくつかの重要な統計情報を確認するためにキャッシュを検査できます。
import com.here.platform.data.client.base.scaladsl.BaseClient
import com.here.platform.data.client.v2.api.scaladsl.versioned.CachingVersionedLayers
import com.here.platform.location.integration.optimizedmap.dcl2.OptimizedMapCatalog
val baseClient: BaseClient = BaseClient()
val optimizedMap: OptimizedMapCatalog =
OptimizedMapCatalog
.from(optimizedMapHRN)
.usingBaseClient(baseClient)
.withMaxBlobCacheSizeBytes(100L * 1024 * 1024)
.withMaxMetadataCacheEntryCount(10000L)
.newInstance
val versionedLayers: CachingVersionedLayers = optimizedMap.versionedLayers
println(versionedLayers.blobCacheStats)
println(versionedLayers.metadataCacheStats)
import com.here.platform.data.client.base.javadsl.BaseClient;
import com.here.platform.data.client.base.javadsl.BaseClientJava;
import com.here.platform.data.client.v2.api.javadsl.versioned.CachingVersionedLayers;
import com.here.platform.location.integration.optimizedmap.dcl2.javadsl.OptimizedMapCatalog;
BaseClient baseClient = BaseClientJava.instance();
OptimizedMapCatalog optimizedMap =
OptimizedMapCatalog.from(optimizedMapHRN)
.usingBaseClient(baseClient)
.withMaxBlobCacheSizeBytes(100 * 1024 * 1024)
.withMaxMetadataCacheEntryCount(10000)
.newInstance();
CachingVersionedLayers versionedLayers = optimizedMap.versionedLayers();
System.out.println(versionedLayers.getBlobCacheStats());
System.out.println(versionedLayers.getMetadataCacheStats());
外部で作成されたキャッシュを再利用します
外部で作成されたキャッシュをさまざまな理由で再使用する必要がある場合があり OptimizedMapLayers
ます。たとえば、事前にいっぱいになったキャッシュを次の場所に渡す場合があります。
import com.here.platform.data.client.base.scaladsl.BaseClient
import com.here.platform.data.client.v2.api.scaladsl.versioned.CachingVersionedLayers
import com.here.platform.data.client.v2.caching.caffeine.scaladsl._
import com.here.platform.location.integration.optimizedmap.OptimizedMapLayers
import com.here.platform.location.integration.optimizedmap.dcl2.{
OptimizedMapCatalog,
OptimizedMapVersionedLayers
}
val baseClient = BaseClient()
val versionedLayerReadersConfiguration = OptimizedMapCatalog.versionedLayerReadersConfiguration(
maxBlobCacheSizeBytes = 100 * 1024 * 1024,
maxMetadataCacheEntryCount = Some(10000))
val blobCache = BlobCacheBuilder(versionedLayerReadersConfiguration).build
val metadataCache = MetadataCacheBuilder(versionedLayerReadersConfiguration).build
val optimizedMapCatalog = OptimizedMapCatalog
.from(optimizedMapHRN)
.usingBaseClient(baseClient)
.withVersionedLayerReadersCache(blobCache, metadataCache)
.newInstance
val optimizedMap: OptimizedMapLayers =
new OptimizedMapVersionedLayers(optimizedMapCatalog.versionedLayers, 42)
val versionedLayers: CachingVersionedLayers = optimizedMapCatalog.versionedLayers
println(versionedLayers.blobCacheStats)
println(versionedLayers.metadataCacheStats)
import com.github.benmanes.caffeine.cache.Cache;
import com.here.platform.data.client.base.javadsl.BaseClient;
import com.here.platform.data.client.base.javadsl.BaseClientJava;
import com.here.platform.data.client.v2.api.javadsl.versioned.CachingVersionedLayers;
import com.here.platform.data.client.v2.caching.caffeine.javadsl.BlobCacheBuilder;
import com.here.platform.data.client.v2.caching.caffeine.javadsl.MetadataCacheBuilder;
import com.here.platform.data.client.v2.caching.javadsl.versioned.VersionedLayerReadersConfiguration;
import com.here.platform.data.client.v2.caching.model.versioned.LayerMetadata;
import com.here.platform.data.client.v2.caching.model.versioned.LayerMetadataKey;
import com.here.platform.data.client.v2.caching.model.versioned.OptionalLayerMetadata;
import com.here.platform.location.integration.optimizedmap.OptimizedMapLayers;
import com.here.platform.location.integration.optimizedmap.dcl2.javadsl.OptimizedMapCatalog;
BaseClient baseClient = BaseClientJava.instance();
VersionedLayerReadersConfiguration versionedLayerReadersConfiguration =
OptimizedMapCatalog.newVersionedLayerReadersConfigurationBuilder()
.withMaxBlobCacheSizeBytes(100 * 1024 * 1024)
.withMaxMetadataCacheEntryCount(10000)
.build();
Cache<LayerMetadata, Object> blobCache =
new BlobCacheBuilder(versionedLayerReadersConfiguration).build();
Cache<LayerMetadataKey, OptionalLayerMetadata> metadataCache =
new MetadataCacheBuilder(versionedLayerReadersConfiguration).build();
OptimizedMapCatalog optimizedMapCatalog =
OptimizedMapCatalog.from(optimizedMapHRN)
.usingBaseClient(baseClient)
.withVersionedLayerReadersCache(blobCache, metadataCache)
.newInstance();
OptimizedMapLayers optimizedMap = optimizedMapCatalog.version(42);
CachingVersionedLayers versionedLayers = optimizedMapCatalog.versionedLayers();
System.out.println(versionedLayers.getBlobCacheStats());
System.out.println(versionedLayers.getMetadataCacheStats());